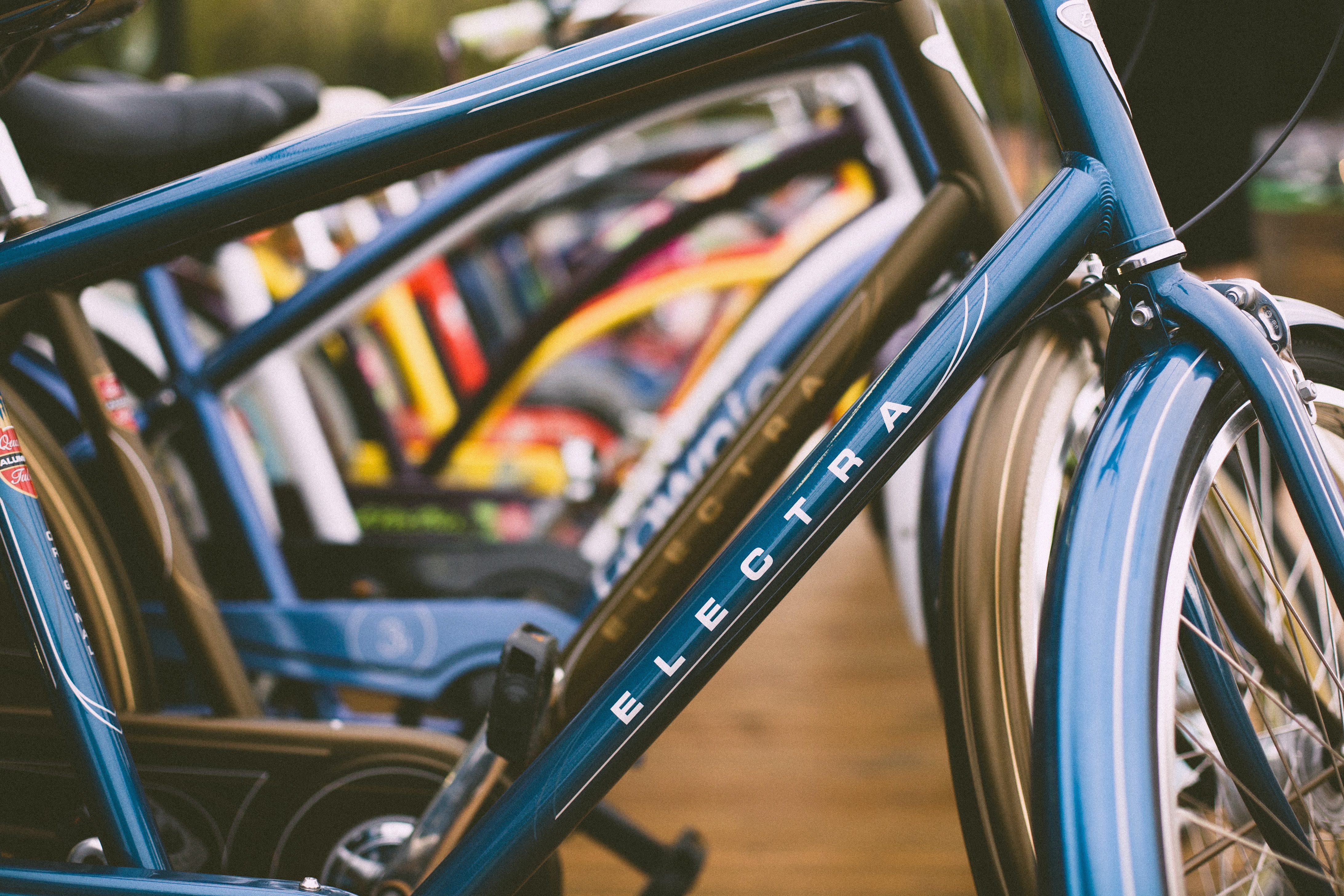
Laravel Collections | Introduction
Laravel Collections are your ultimate weapon for wrangling and manipulating data in the most elegant and efficient way possible. These versatile collections are your personal data wizards, allowing you to perform a variety of operations conveniently. Whether you're dealing with database results, API responses, or simple arrays, Laravel Collections provide a consistent and expressive interface that simplifies your code and elevates your data-handling game.
In this series of articles, we'll explore the power and versatility of Laravel Collections. I have divided this series into the following articles, from time to time, I will update the series accordingly:
Part 1:
- all
- average
- avg
- chunk
- chunkWhile
- collapse
- collect
- combine
- concat
- contains
- containsOneItem
- containsStrict
- count
- countBy
- crossJoin
Part 2:
- dd
- diff
- diffAssoc
- diffAssocUsing
- diffKeys
- doesntContain
- dot
- dump
- duplicates
- duplicatesStrict
- each
- eachSpread
- ensure
- every
- except
Part 3:
- filter
- first
- firstOrFail
- firstWhere
- flatMap
- flatten
- flip
- forget
- forPage
- get
- groupBy
- has
- hasAny
- implode
- intersect
Part 4:
- intersectAssoc
- intersectByKeys
- isEmpty
- isNotEmpty
- join
- keyBy
- keys
- last
- lazy
- macro
- make
- map
- mapInto
- mapSpread
- mapToGroups
Part 5:
- mapWithKeys
- max
- median
- merge
- mergeRecursive
- min
- mode
- nth
- only
- pad
- partition
- percentage
- pipe
- pipeInto
- pipeThrough
Part 6:
- pluck
- pop
- prepend
- pull
- push
- put
- random
- range
- reduce
- reduceSpread
- reject
- replace
- replaceRecursive
- reverse
- search
Part 7:
- shift
- shuffle
- skip
- skipUntil
- skipWhile
- slice
- sliding
- sole
- some
- sort
- sortBy
- sortByDesc
- sortDesc
- sortKeys
- sortKeysDesc
Part 8:
- sortKeysUsing
- splice
- split
- splitIn
- sum
- take
- takeUntil
- takeWhile
- tap
- times
- toArray
- toJson
- transform
- undot
- union
Part 9:
- unique
- uniqueStrict
- unless
- unlessEmpty
- unlessNotEmpty
- unwrap
- value
- values
- when
- whenEmpty
- whenNotEmpty
- where
- whereStrict
- whereBetween
- whereIn
Part 10:
- whereInStrict
- whereInstanceOf
- whereNotBetween
- whereNotIn
- whereNotInStrict
- whereNotNull
- whereNull
- wrap
- zip