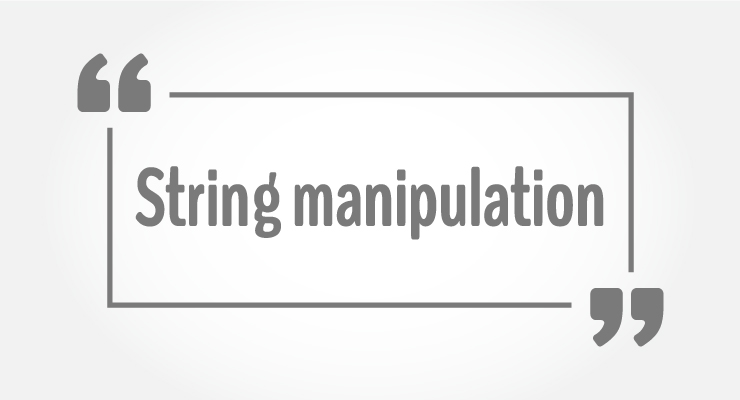
Laravel: String manipulations with 'Str' class
Laravel already provides powerfull helpers for string manipulations, but I was wondering what's behind these convinient helpers. The Laravel string helpers is powered by Illuminate\Support\Str
class. Let's explore what methods it contains and how we can use them. For this example I will be using 'Lorem Ipsm ....' for subject testing. Here's the list of all the methods used, you can jump right to your desired method from here:
- after()
- ascii()
- before()
- camel()
- contains()
- endsWith()
- finish()
- is()
- kebab()
- length()
- limit()
- lower()
- words()
- plural()
- random()
- replaceArray()
- replaceFirst()
- replaceLast()
- start()
- upper()
- title()
- singular()
- slug()
- snake()
- startsWith()
- studly()
- substr()
- ucfirst()
1- after($subject, $search) : string
This method chops off all the characters including $search
from the start in the $subject
and returns the rest of the string. Let's test this method:


2- ascii($value, $language = 'en') : string
This method returns the ascii equivalent of the $value
string in the $language
specified.

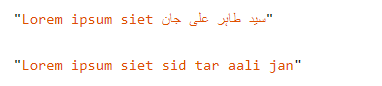
3- before($subject, $search) : string
This is opposite of after()
method. This will only keep the characters before the$search
characters:

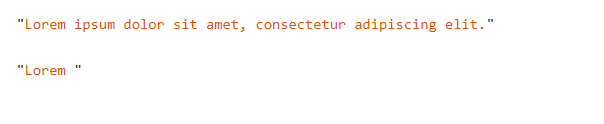
4- camel($value) : string
This method will return a camel-case string. It will remove any spaces and join the words as shown below:


5- contains($haystack, $needles) : bool
This method returns boolean true
or false
if a string $haystack
contains a string / characters $needles
. This is a case-sensitive method, means if it has a work 'tomorrow', it will return false
for 'Tomorrow' as demonstrated below:
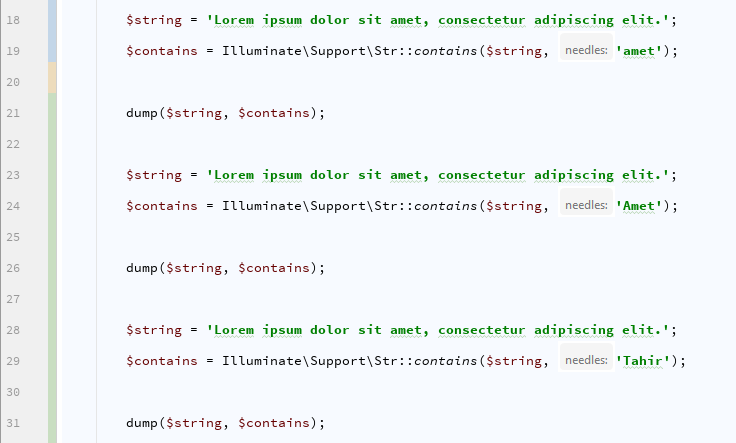
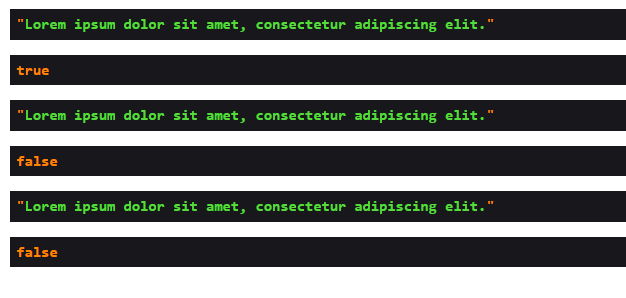
6- endsWith($haystack, $needles) : bool
This method tests if $haystack ends with $needles which can be a string or an array. Let's check the example below:
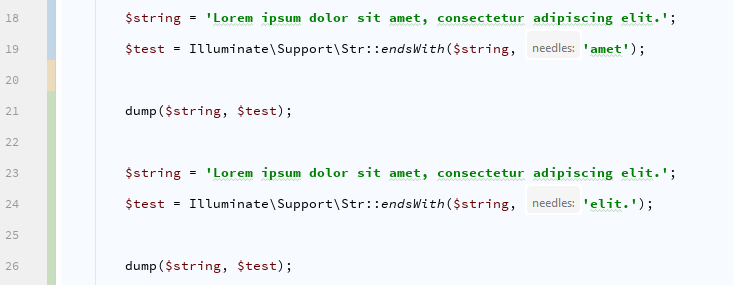
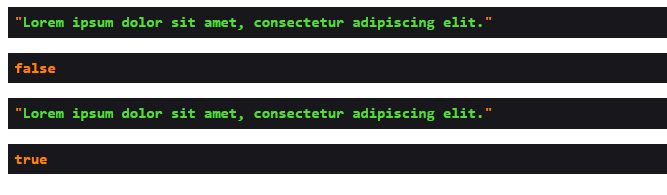
7- finish($value, $cap) : string
This method add a padding to the end of the string. In other words, right-pad the string, as shown below:


8- is($pattern, $value) : bool
This method tests $value
against the $pattern
which can be a string
or an array
of strings and returns true or false if the string contains the pattern. As shown below in the example:
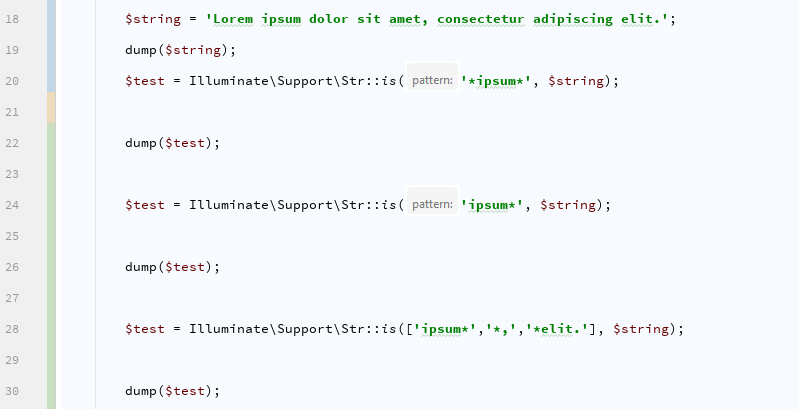
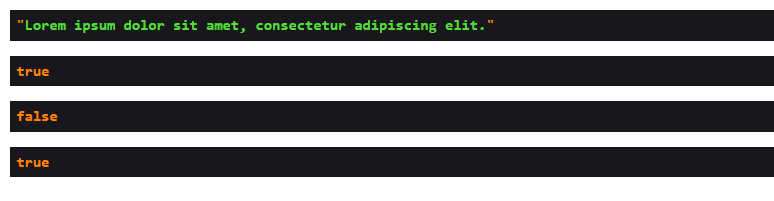
9- kebab($value) : string
This method turns your string into a kebab-case string.


10- length($value, $encoding = null) : int
This method uses mbstring extension's mb_strlen() function for length, for non-ascii (Unicode) strings you have to provide $encoding
scheme name such as UTF-8, UTF-16 etc.

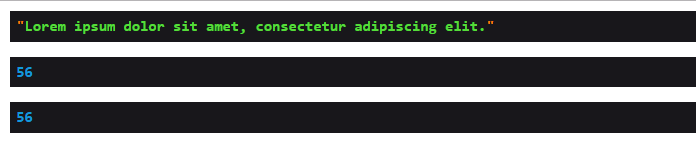
11- limit($value, $limit = 100, $end = '...') : string
This method limits the string at a $limit
, the default value for limit is 100
characters which is excluding the $end
text.


12- lower($value) : string
This method converts the string to a lower-case string.


13- words($value, $words = 100, $end = '...') : string
This method limits the string on words just like limit() method.


14- plural($value, $count = 2) : string
This method converts a given word or last word of the string to plural form.
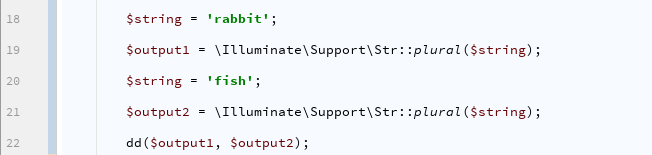
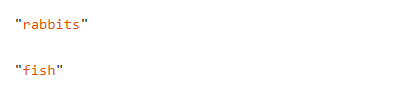
15- random($length = 16) : string
This method generates a random string, the default length of the returned string is 16.


16- replaceArray($search, array $replace, $subject)
This method replaces all the instances of $search in $subject with $replace in a starting order, you will get more clarity with the following example:
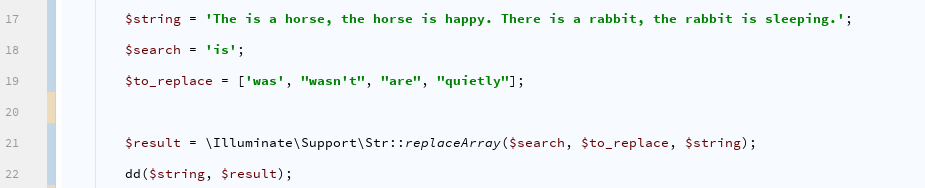

17- replaceFirst($search, $replace, $subject) : string
This method will only replace the first occurance of $search
with $replace
in the $subject
string as shown in the example below:
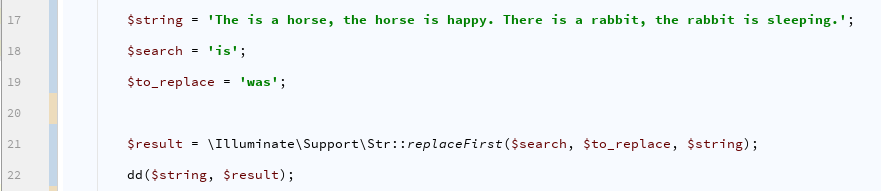

18- replaceLast($search, $replace, $subject) : string
Like replaceFirst()
this method replaces the last occurance of $search
with $replace
in the $subject
string.
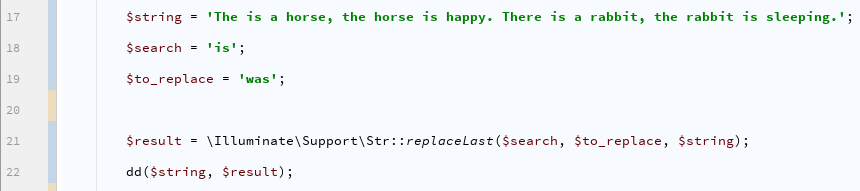

19- start($value, $prefix) : string
This method adds $prefix
to the starting of the $value
. In other words it left-pads the string.


20- upper($value) : string
This method converts $value
to upper-case string. It uses mb_strtoupper()
.


21- title($value) : string
This method converts $value
to title-case string.

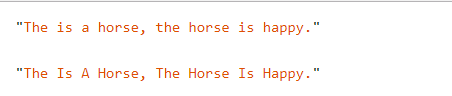
22- singular($value) : string
Opposite ofplural()
method, it converts plural word $valule
into a singular word.


23- slug($title, $separator = '-', $language = 'en') : string
Turns given string into a slug-case string which can be useful for SEO friendly urls. It will remove any non-printable characters, special characters and convert unicode into ascii characters.
By default, the separator used is '-' but you can specify your own, like I used a '+' sign:
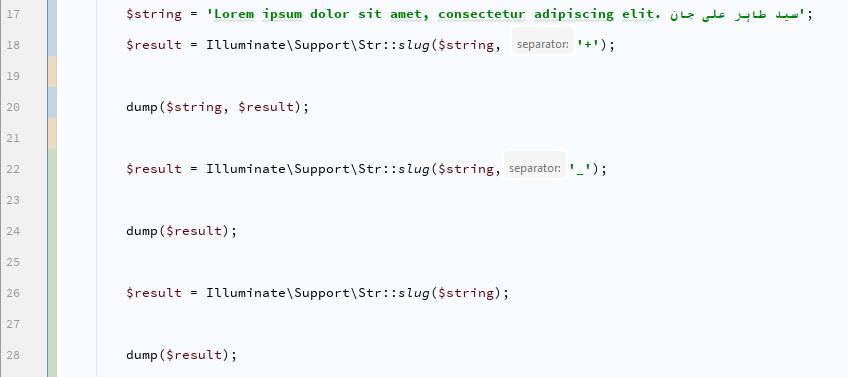
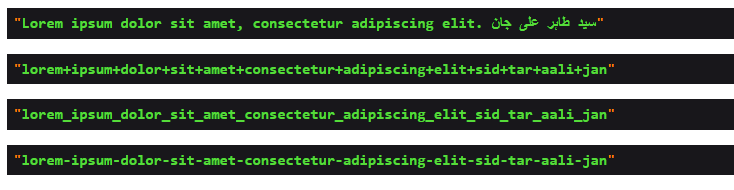
24- snake($value, $delimiter = '_') : string
This method will convert the string $value
into a snake-case string with $delimiter
specified, an _ will be used if unspecified.
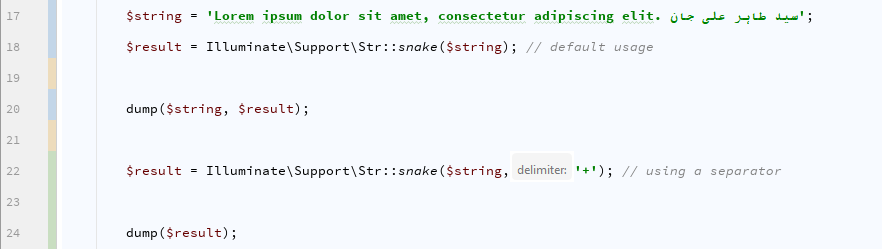

25- startsWith($haystack, $needles) : bool
This method tests if a given string $haystack
starts with a string or one of strings in the array $needles
.
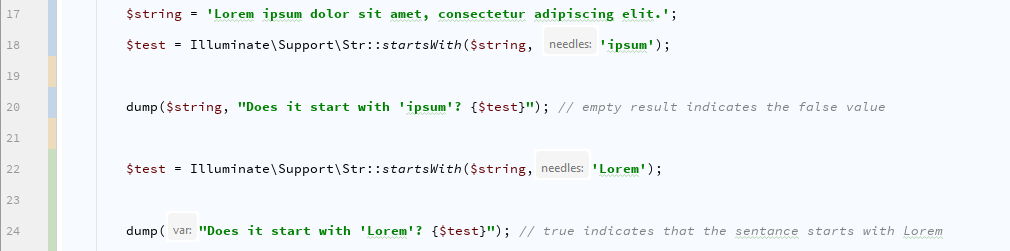
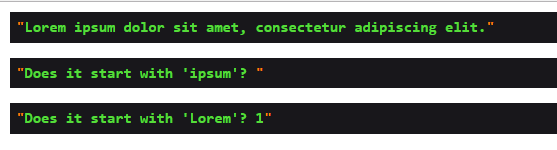
26- studly($value) : string
This method will return a studly-case string of $value
.


27- substr($string, $start, $length = null) : string
This method returns a sub-string of a $string
from position $start
, $length
is optional but you can specify to return the number of characters to be return, it will return the rest of the string if not specified.
This method uses mbstring's mb_substr()
function:
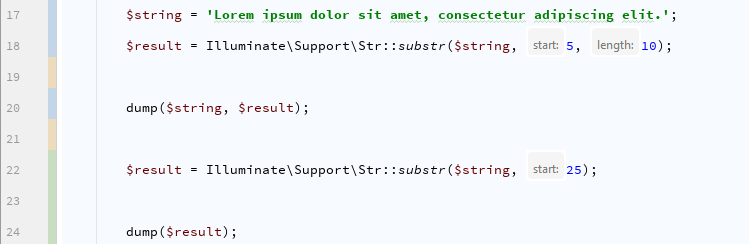
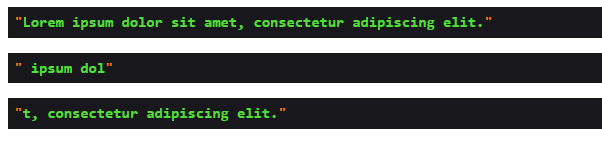
28- ucfirst($string) : string
I don't know how this method compares to the PHP native ucfirst() function in terms of speed and memory usage but it's there. This method capitalizes the first character / letter of the string given.

