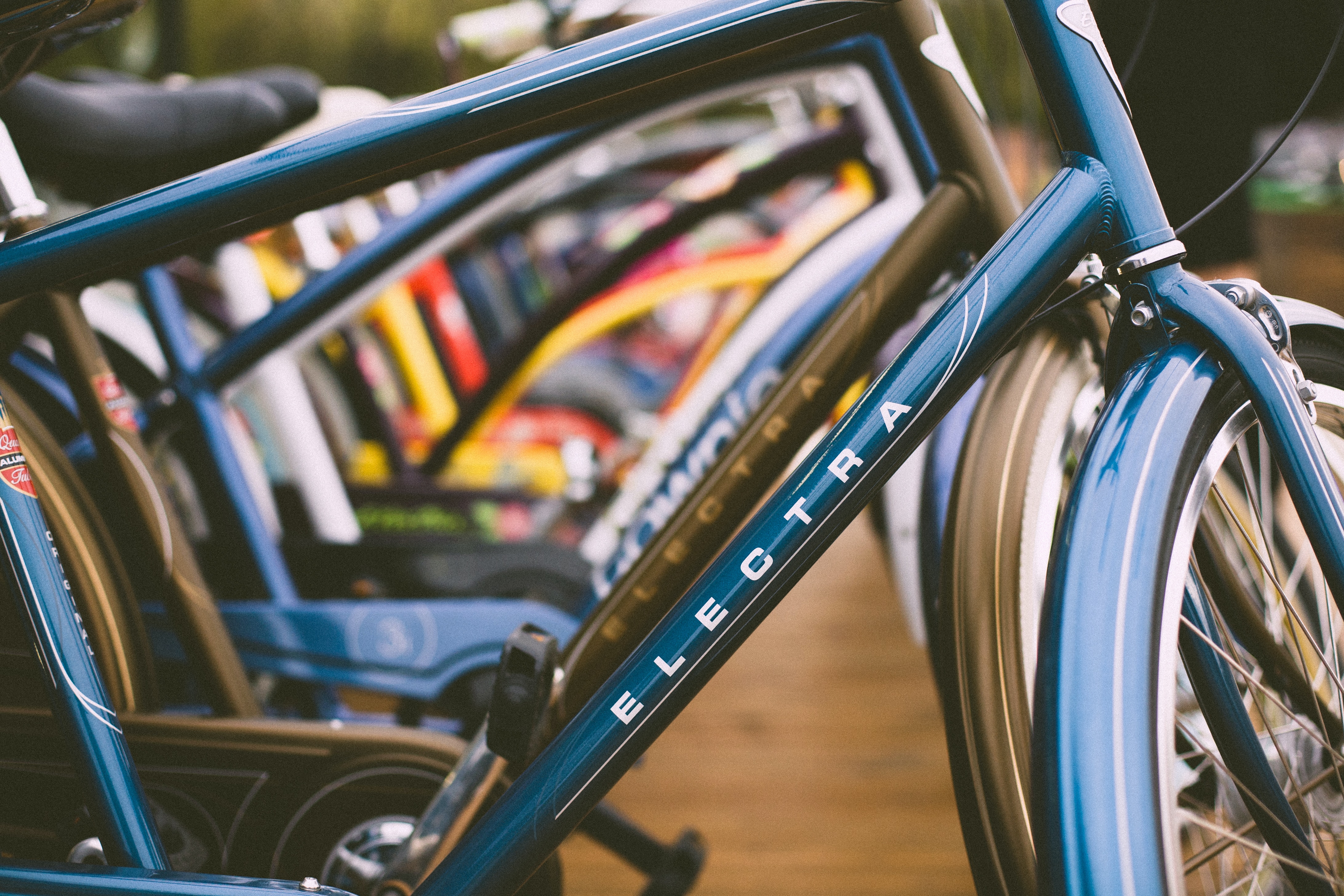
Laravel Collections | Part 1
In Part 1, we'll delve into a variety of Collection methods, each accompanied by an example:
1. all()
The all
method returns all the items from the collection as a plain array. It's a straightforward way to convert a collection into a standard PHP array.
$collection = collect([1, 2, 3]);
$array = $collection->all();
// Result: [1, 2, 3]
2. average()
The average
method calculates the average of all numeric values in the collection. It's handy when you need to find the mean value of a set of numbers.
$collection = collect([1, 2, 3, 4, 5]);
$average = $collection->average();
// Result: 3.0
3. avg()
Similar to average()
, the avg
method calculates the average of all numeric values in the collection. It provides an alias for the same functionality.
$collection = collect([1, 2, 3, 4, 5]);
$average = $collection->avg();
// Result: 3.0
4. chunk()
The chunk
is a very handy method, it breaks the collection into smaller chunks of a specified size. It's useful for paginating large datasets or processing data in batches.
$collection = collect([1, 2, 3, 4, 5, 6, 7, 8]);
$chunks = $collection->chunk(3);
// Result: [[1, 2, 3], [4, 5, 6], [7, 8]]
5. chunkWhile()
The chunkWhile
method allows you to chunk a collection based on a callback function's logic. It chunks the data until the callback returns false
.
$collection = collect([1, 2, 3, 4, 5, 6]);
$chunks = $collection->chunkWhile(fn($item) => $item % 2 === 0);
// Result: [[1], [2, 3], [4, 5], [6]]
6. collapse()
The collapse
method flattens a multi-dimensional collection into a single-dimensional one. It's handy when you have nested arrays and want to simplify the structure.
$collection = collect([[1, 2], [3, 4], [5, 6]]);
$collapsed = $collection->collapse();
// Result: [1, 2, 3, 4, 5, 6]
7. collect()
The collect
function is not a method but a global helper function that converts an array into a collection instance.
$array = [1, 2, 3];
$collection = collect($array);
// Now, $collection is an instance of Illuminate\Support\Collection
8. combine()
The combine
method combines the values of one collection as keys with the values of another collection as values to create a new collection.
$keys = collect(['name', 'age']);
$values = collect(['John', 30]);
$combined = $keys->combine($values);
// Result: ['name' => 'John', 'age' => 30]
9. concat()
The concat
method appends the items of another array or collection to the current collection, creating a new collection.
$collection = collect([1, 2]);
$concatenated = $collection->concat([3, 4]);
// Result: [1, 2, 3, 4]
10. contains()
The contains
method checks if the collection contains a specific value. It returns true
if the value is found, otherwise false
.
$collection = collect(['apple', 'banana', 'cherry']);
$containsBanana = $collection->contains('banana');
// Result: true
11. containsOneItem()
The containsOneItem
method checks if the collection contains only one item. It returns true
if there's exactly one item in the collection.
$collection = collect(['apple']);
$containsOne = $collection->containsOneItem();
// Result: true
12. containsStrict()
The containsStrict
method checks if the collection contains a specific value using strict comparison (===). It returns true
if the value is found strictly, otherwise false
.
$collection = collect([1, '1']);
$containsStrict = $collection->containsStrict(1);
// Result: true
13. count()
The count
method returns the number of items in the collection.
$collection = collect([1, 2, 3, 4, 5]);
$count = $collection->count();
// Result: 5
14. countBy()
The countBy
method groups the collection's items by a key or callback function and returns the count of items in each group as a new collection.
$collection = collect(['apple', 'banana', 'cherry']);
$counted = $collection->countBy(fn($item) => strlen($item));
// Result: [5 => 2, 6 => 1]
15. crossJoin()
The crossJoin
method returns all possible combinations of the items from multiple arrays or collections, creating a new collection of arrays.
$letters = collect(['A', 'B']);
$numbers = collect([1, 2]);
$combinations = $letters->crossJoin($numbers);
// Result: [['A', 1], ['A', 2], ['B', 1], ['B', 2]]
In Part 2, we'll explore more of what Laravel Collections has to offer, enabling you to become a true Collection master in your Laravel projects. Stay tuned and Happy Coding!