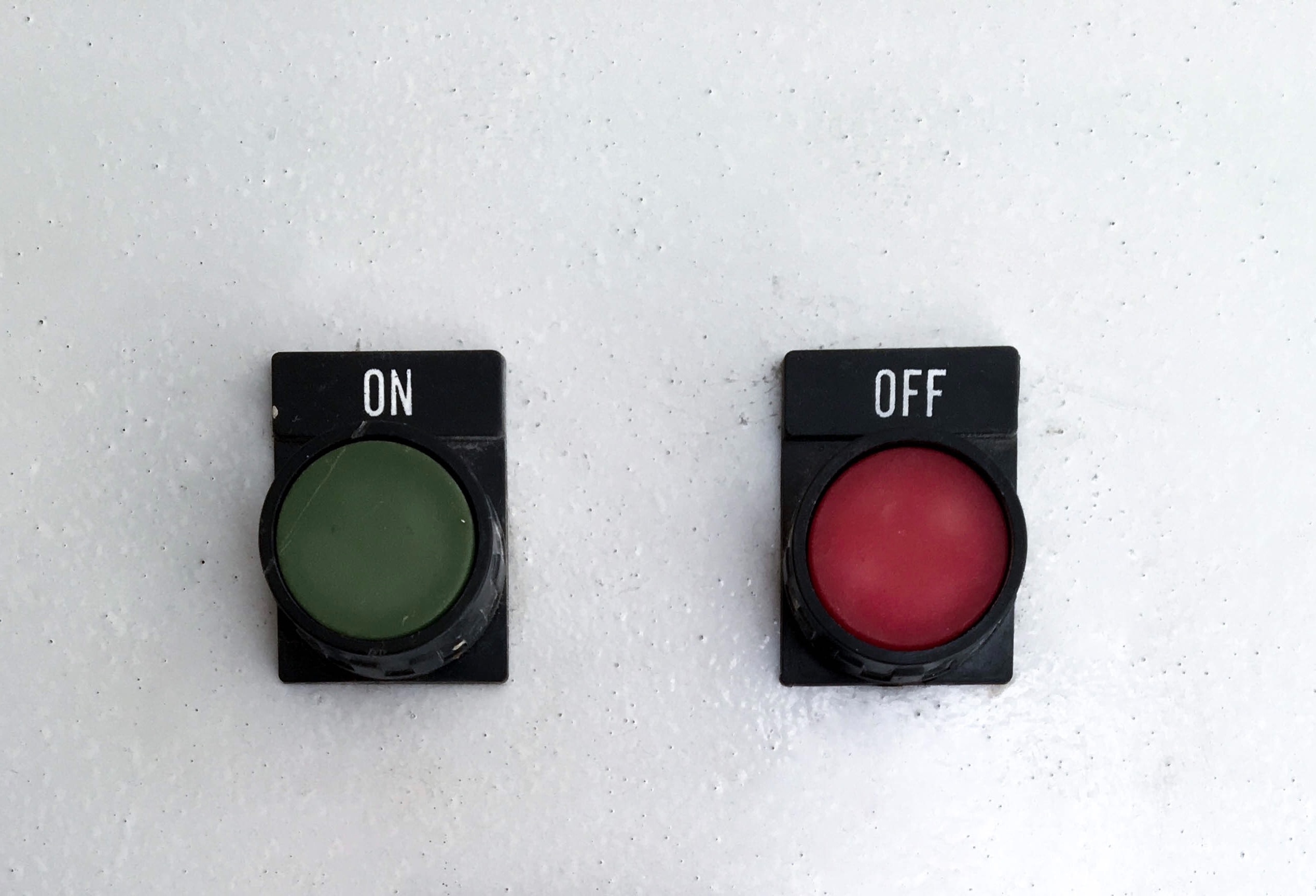
Event driven Development in Laravel
In the world of modern web development, creating interactive and responsive applications is key to providing a seamless user experience. Laravel, a popular PHP framework, offers a robust foundation for building such applications. One powerful concept that Laravel brings to the table is event-based development. In this article, we'll explore how to harness the power of events in Laravel by creating a real-world example: ordering food from an online restaurant menu.
What are Events in Laravel?
Events in Laravel allow you to create and handle custom events within your application. These events can be triggered at specific points in your code and can be listened to by one or more event listeners. Event-based development follows the Publish-Subscribe pattern, where publishers emit events, and subscribers listen for and react to those events.
Setting Up the Laravel Project
Before we dive into the code, make sure you have Laravel installed on your system. If not, you can follow the installation instructions on the Laravel website.
Let's start by creating a new Laravel project named "Restaurant
."
laravel new Restaurant
Now, navigate to your project's root folder and open it in your favorite code editor.
Creating the Menu
In our food ordering example, we'll begin by creating a menu of delicious items. We'll define these items as an array in our code.
// routes/web.php
Route::get('/', function () {
$menu = [
'Burger' => 10,
'Pizza' => 12,
'Pasta' => 8,
'Salad' => 6,
];
return view('menu', ['menu' => $menu]);
});
Here, we define a route that renders a view named "menu
" and passes an array of food items with their prices.
Creating the Menu View
Next, we'll create the menu view that displays the available food items and allows users to place an order.
<!-- resources/views/menu.blade.php -->
<!DOCTYPE html>
<html>
<head>
<title>Food Menu</title>
</head>
<body>
<h1>Food Menu</h1>
<ul>
@foreach($menu as $item => $price)
<li>
{{ $item }} - ${{ $price }}
<form method="POST" action="{{ route('order', $item) }}">
@csrf
<button type="submit">Order</button>
</form>
</li>
@endforeach
</ul>
</body>
</html>
In this view, we iterate over the menu items, display their names and prices, and provide an "Order" button for each item. The form associated with each item will submit a POST request to the "order" route with the item name as a parameter. I know this looks bad but bear with me for this simple example.
Defining the Event
Now, let's define an event that represents an order being placed. We'll call it "FoodOrderedEvent
."
php artisan make:event FoodOrderedEvent
This command generates a new event class in the "app/Events
" directory. Open the "FoodOrderedEvent.php
" file and add a public property to hold the ordered item.
<?php
// app/Events/FoodOrderedEvent.php
namespace App\Events;
use ...
class FoodOrderedEvent
{
use Dispatchable, InteractsWithSockets, SerializesModels;
public string $item;
public function __construct(string $item)
{
$this->item = $item;
}
...
}
Creating an Event Listener
Next, we'll create an event listener to handle the food orders. Run the following command to generate an event listener.
php artisan make:listener OrderListener
Open the "OrderListener.php
" file in the "app/Listeners" directory. In the "handle" method, we'll simulate processing the order by logging a message.
<?php
// app/Listeners/OrderListener.php
namespace App\Listeners;
use App\Events\FoodOrderedEvent;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Queue\InteractsWithQueue;
class OrderListener
{
public function handle(FoodOrderedEvent $event)
{
$item = $event->item;
Log::info("Order placed for $item.");
}
}
Registering the Event Listener
To ensure that our event listener is registered and listening for events, open the "EventServiceProvider.php
" file in the "app/Providers
" directory. Add the following line to the "$listen
" array:
<?php
// app/Providers/EventServiceProvider.php
namespace App\Providers;
use Illuminate\Auth\Events\Registered;
use Illuminate\Auth\Listeners\SendEmailVerificationNotification;
use Illuminate\Foundation\Support\Providers\EventServiceProvider as ServiceProvider;
use Illuminate\Support\Facades\Event;
class EventServiceProvider extends ServiceProvider
{
protected $listen = [
FoodOrderedEvent::class => [
OrderListener::class,
],
Registered::class => [
SendEmailVerificationNotification::class,
],
];
....
}
This configuration tells Laravel to listen for "FoodOrderedEvent
" events and handle them using the "OrderListener
" listener.
Triggering the Event
Now, we need to trigger the "FoodOrderedEvent
" event when a user places an order. Update the "web.php
" file to define a route that handles food orders.
// routes/web.php
Route::post('/order/{item}', function ($item) {
event(new FoodOrderedEvent($item));
return redirect('/');
})->name('order');
In this route, we use the "event" helper function to fire the "FoodOrderedEvent
" event with the selected item as a parameter. After triggering the event, we redirect the user back to the menu.
Testing the Application
Finally, you can start your Laravel development server and visit http://localhost:8000
to explore the food menu and place orders. Each time an order is placed, the event listener will log a message in the Laravel log files.
php artisan serve
Conclusion
Event-based development in Laravel provides a powerful way to decouple components of your application and handle various tasks asynchronously. In this example, we've seen how to create a simple food ordering system using Laravel events. You can extend this concept to build more complex and interactive applications with ease. Events are a fundamental tool in Laravel's toolkit, enabling you to create elegant and responsive web applications. Happy coding!