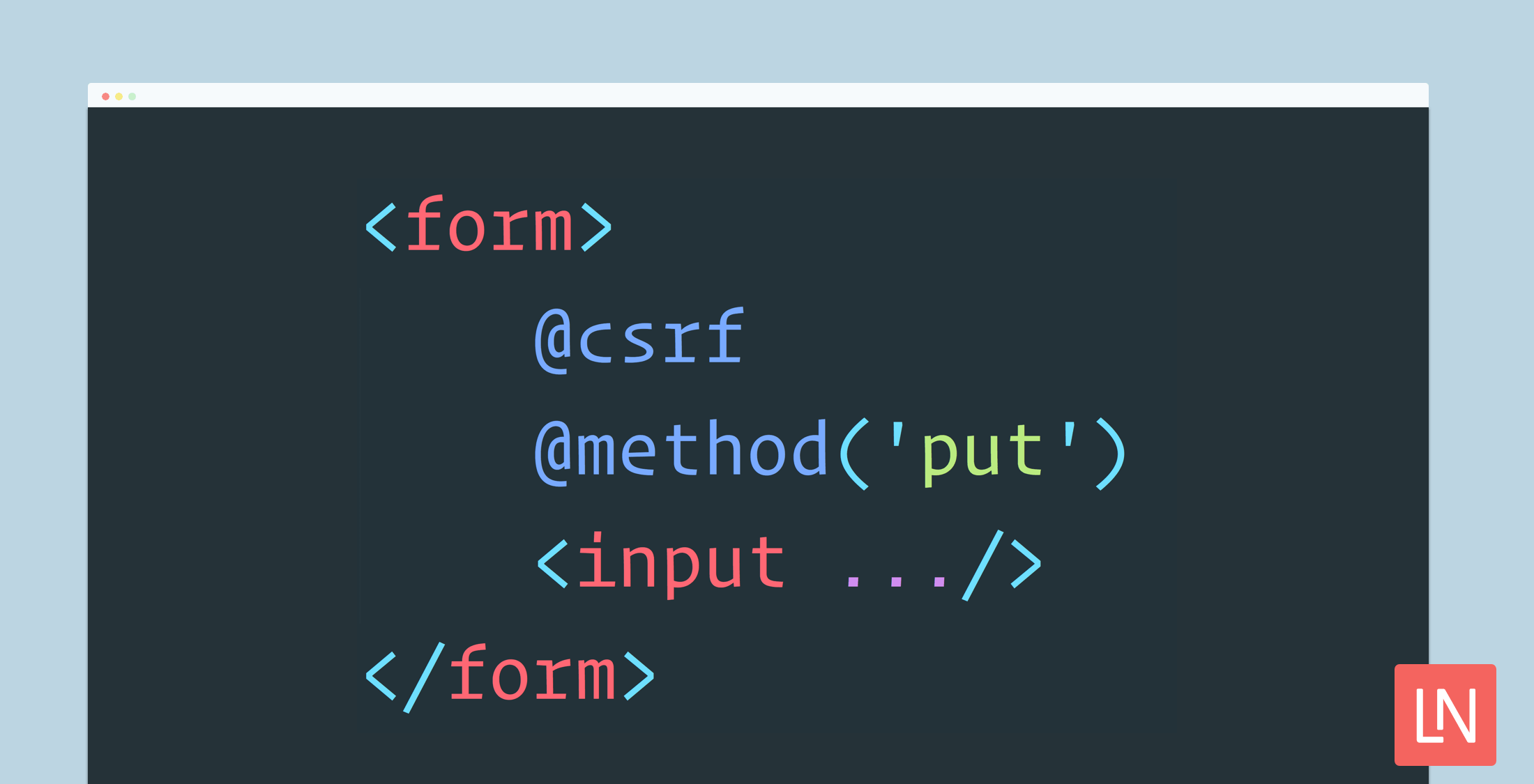
Laravel: Add @method() & @csrf to Blade
Taylor Otwell tweeted about his plans to add @method()
and @csrf
. I'm currently using Laravel 5.5 so I have to add these to a ServiceProvider
to make use of it. This tutorial discusses how to add such helpers to your edition of Laravel.
Let's start right off from creating a ServiceProvider
:
php artisan make:provider BladeServiceProvider
or just create a class called BladeServiceProvider
in your app\Providers
directory and add the following code:
<?php
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
class BladeServiceProvider extends ServiceProvider
{
/**
* Bootstrap the application services.
*
* @return void
*/
public function boot()
{
//
}
/**
* Register the application services.
*
* @return void
*/
public function register()
{
//
}
}
Now let's add logic for @method
in the boot method of BladeServiceProvider
Blade::directive('method', function ($method)
{
return '<input type="hidden" name="_method" value="<?php echo strtoupper(' . $method . '); ?>">';
});
Now let's add logic for @csrf
helper like above.
Blade::directive('csrf', function ()
{
return '<?php echo csrf_field(); ?>';
});
Your code should look similar to the following:
<?php
namespace App\Providers;
use Blade;
use Illuminate\Support\ServiceProvider;
class BladeServiceProvider extends ServiceProvider
{
/**
* Bootstrap the application services.
*
* @return void
*/
public function boot()
{
Blade::directive('csrf', function ()
{
return '<?php echo csrf_field(); ?>';
});
Blade::directive('method', function ($method)
{
return '<input type="hidden" name="_method" value="<?php echo strtoupper(' . $method . '); ?>">';
});
}
/**
* Register the application services.
*
* @return void
*/
public function register()
{
//
}
}
Now add BladeServiceProvider::class
to the providers
array in your config\app.php
file like this:
'providers' => [
...
App\Providers\BladeServiceProvider::class,
...
Now finally, you can add @method()
and @csrf
in Blade template conveniently like this:
<form action="..." class="...">
@csrf
@method('put')
@hidden('user_id',$user->id)<input type="first_name" name="first_name">
</form>
Thanks for reading!